To get up to speed, make sure you check out part 1:
In this part of the tutorial we are going to add the functionality of deleting posts and refresh our feed. In order to achieve this, we want to make sure that we apply an action to our more button within our Post Cell. So, let’s first assign a target action to our optionsBtn in our Post Cell class. When we press our optionsBtn, the optionsBtnPressed() function is going to be called, which we also have to implement.
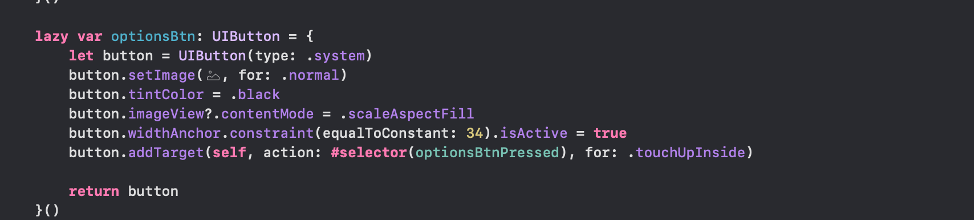

Now we want to present a UIAlertController from our HomeController class to be able to manipulate our Post Cell data. We can achieve this by implementing a delegate, which I will call PostCellHandleOptionsDelegate. I am going to implement this delegate in my HomeController.swift file to make things easier for now.

We want our delegate to have a function that will be implemented by our HomeController class to handle the presentation of our UIAlertController. I will call this function handleOptions(cell: PostCell). This function takes in a PostCell as an argument, so we can identify the cell to delete in our HomeController class.

Great! Now let’s add a reference to this delegate in our PostCell class and fire off its handleOptions(…) property when the user clicks on the optionsBtn.


Now we let’s make our HomeController class conform to this delegate via an extension to make our code a bit cleaner.

Now in this function, we will handle the instantiation of our UIAlertController, as well as giving it the option of being able to delete the corresponding cell, and the option to cancel the action.
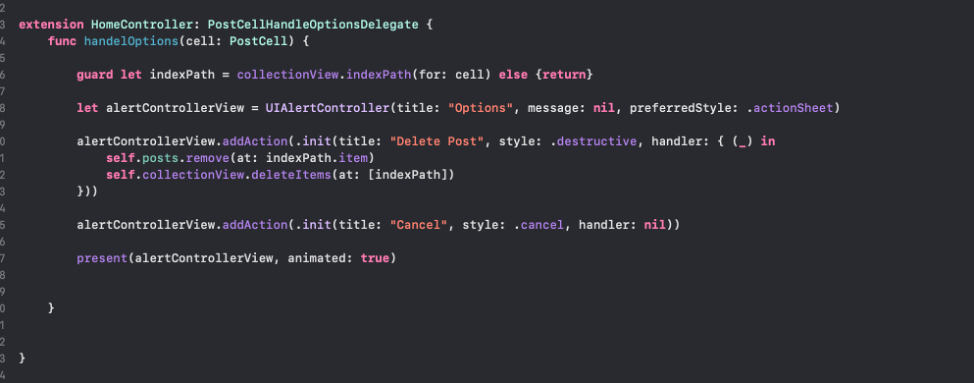
Awesome, almost done! Now we need to let our cell know that the HomeController class is the class that will be conforming to the delegate.
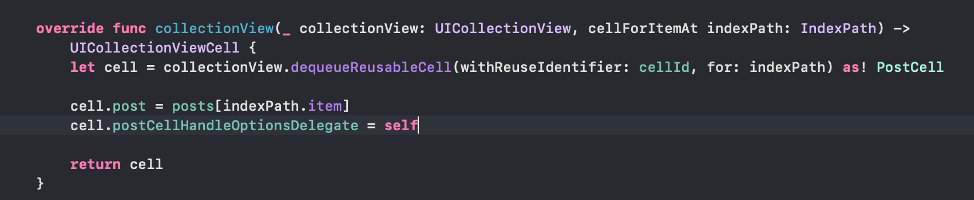
Great job! Now you can delete post cells.
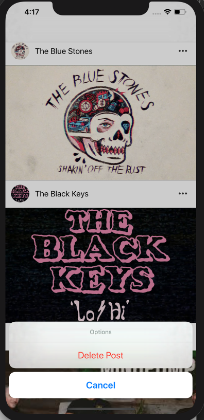
Now let’s give our collection view a refresh controller to be able to retrieve our deleted post cells. We will do this in out HomeController class’ viewDidLoad(). In addition to this we will have to provide our fetchPosts() with a slight modification.

There you have it folks! To make our code base a lot cleaner and avoid the massive-view-controller issued, you can place each class in a file and have a folder structure like the following:
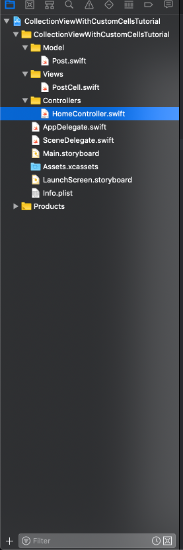
If you made it this far, thanks for checking out this tutorial. This tutorial was inspired by Brian Voong’s fullstack social app. Let me know if there is an app feature you would like to see me implement. Cheers! You can get the full project here:
https://github.com/gabedelvillar/CollectionViewWithCustomCellsTutorial-iOS
Leave a Reply